Multiple Choice Identify the
choice that best completes the statement or answers the question.
|
|
1.
|
What must be written on line 12 in order to run this program? 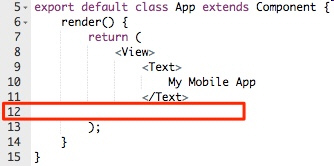
a. | <Text> | c. | <View> | b. | </Text> | d. | </View> |
|
|
2.
|
Twelve identical circles fit inside the rectangle as shown below. If the
rectangle has a width of 24 units and a height of 18 units, what is the radius of each
circle? 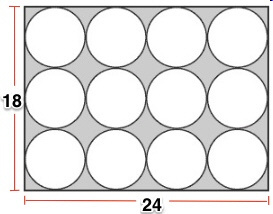
|
|
3.
|
Consider the following program code, where ‘hour’ is an input from
the user.
if(hour <= 10){ println("Good morning!"); }
else if(hour < 16){ println("Good afternoon!"); } else
{ println("Hello!"); } Of the following choices, select the
value for ‘hour’ that would cause this program to print “Good
Afternoon!”
a. | 8 | c. | 18 | b. | 14 | d. | “Good
afternoon!” |
|
|
4.
|
The image below shows a rectangle that is split into equal parts. If the width
of the entire rectangle is 25 units, what is the width of one of the smaller rectangles? 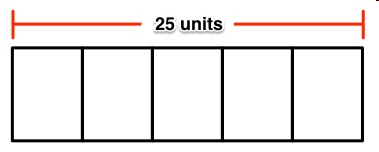
|
|
5.
|
Jennifer will wear a coat if it is raining or if it is less than 50°F
outside. Otherwise, Jennifer will leave her coat at home. In which of the following cases will
Jennifer leave her coat at home?
a. | It is raining and 30°F | c. | It is not raining and
45°F | b. | It is raining and 55°F | d. | It is not raining and 50°F |
|
|
6.
|
Which of the following style rules is syntactically correct and uses the best
style practices?
a. | button: { backgroundColor: yellow
alignItems: ‘center’ } | c. | BUTTON = { backgroundColor=
‘yellow’, alignItems= ‘center’ } | b. | Button =
{ backgroundColor: yellow alignItems:
‘center’ } | d. | button: { backgroundColor:
‘yellow’, alignItems:
‘center’ } |
|
|
7.
|
What would need to go inside the parent view container in order to have the
small dark blue square inside the large light blue square in the program below? 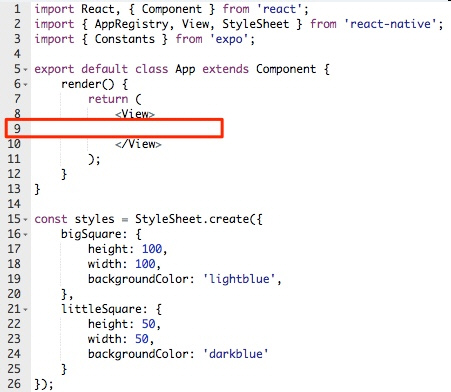
a. | <View style={styles.littleSquare}> <View
style={styles.bigSquare}> </View> </View> | c. | <View
style={styles.bigSquare}> <View
style={styles.littleSquare}>
</View> </View> | b. | <View
style={styles.bigSquare}> </View> <View
style={styles.littleSquare}> </View> | d. | <View
style={styles.littleSquare}> </View> <View
style={styles.bigSquare}> </View> |
|
|
8.
|
The following program is being written to keep track of which team is taking
their turn. The red team will be up first, with the word “Red” showing on the screen.
When the ‘Next team up!’ button is clicked, the text should change to read
“Blue”. 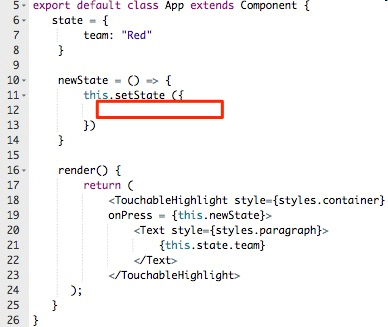 What should be written on line 12 to change the
text to read “Blue” when clicked?
a. | team: Blue | c. | newTeam: “Blue” | b. | team:
“Blue” | d. | team=
Blue |
|
|
9.
|
The following program draws 3 stripes on the screen, with the orange stripes
half the height of the green stripe, as shown in the image. How could the StyleSheet be altered to
have the stripes fill the screen no matter the screen size? import React, { Component } from
'react'; import { AppRegistry, View, StyleSheet } from
'react-native'; import { Constants } from 'expo'; export default class
App extends Component { render()
{ return
( <View
style={styles.container}>
<View style={styles.smallStripe}>
</View>
<View style={styles.largeStripe}>
</View>
<View style={styles.smallStripe}>
</View>
</View> );
} } const styles = StyleSheet.create({ largeStripe:
{ height:
100, backgroundColor:
'green' }, smallStripe:
{ height:
50, backgroundColor:
'orange' } }); 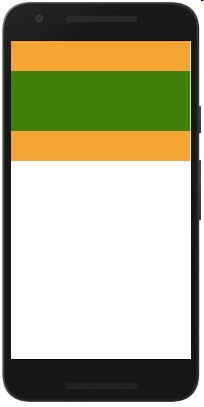
a. | largeStripe: { height: 500,
backgroundColor: ‘green’, }, smallStripe: { height:
250, backgroundColor: ‘orange’ } | c. | largeStripe:
{ flex: 2, backgroundColor:
‘green’, }, smallStripe: { flex: 1,
backgroundColor: ‘orange’ } | b. | largeStripe: { height:
100, flex: 1, backgroundColor:
‘green’, }, smallStripe: { height: 250,
flex: 2, backgroundColor: ‘orange’ } | d. | largeStripe: {
backgroundColor: ‘green’, }, smallStripe: { backgroundColor:
‘orange’ } |
|
|
10.
|
Which of the following pieces of code is the correct way to create a button that
will show the text ‘YOU PRESSED ME!’ when clicked?
a. | export default class App extends Component { render()
{ return
( <View
style={styles.container}>
<View style={styles.button}>
<TouchableHighlight
onPress = {() =>
{
alert('YOU PRESSED
ME!')
}}
>
</TouchableHighlight>
</View>
</View> );
} } | c. | export default class App extends Component { render()
{ return
( <View
style={styles.container}>
<TouchableHighlight
onPress = {() =>
{
alert('YOU PRESSED
ME!')
}}
>
<View style={styles.button}>
</View>
</TouchableHighlight>
</View> );
} } | b. | export default class App extends Component { render()
{ return
( <View
style={styles.container}>
<TouchableHighlight>
<View style={styles.button}>
YOU PRESSED
ME!
</View>
</TouchableHighlight>
</View> );
} } | d. | export default class
App extends Component { render()
{ return
( <View
style={styles.container}>
<View style={styles.button}>
<TouchableHighlight>
YOU PRESSED
ME!
</TouchableHighlight>
</View>
</View> );
} } |
|
|
11.
|
What does a TouchableHighlight do? I. Makes a text component clickable II.
Makes a view component clickable III. Highlights a button
a. | I | c. | III | b. | II and III | d. | I and II |
|
|
12.
|
What should be written on line 14 to print the text saved inside the variable
‘name’? 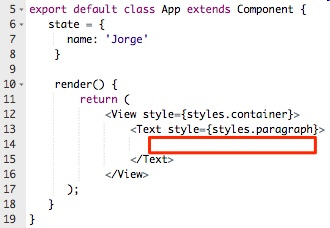
a. | {this.state.name} | c. | {name} | b. | {state.name} | d. | this.name |
|
|
13.
|
The following program is written to keep track of the innings in a baseball
game. 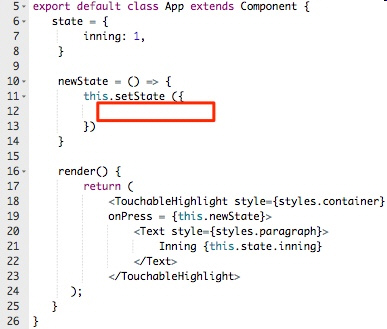 What must be written on line 12 to correctly
update the inning state to count by 1’s?
a. | inning = inning + 1 | c. | inning = {this.state.inning} + 1 | b. | inning: inning +
1 | d. | inning: this.state.inning
+ 1 |
|
|
14.
|
The following render function is used to create an app that will change a text
string to upper or lowercase letters based on the button that is clicked. render()
{ return ( <View
style={styles.container}>
<TouchableHighlight
style={styles.button}>
onPress = {this.uppercase}>
<Text style={styles.buttonText}>
Uppercase
</Text>
</TouchableHighlight>
<Text style={styles.paragraph}>
{this.state.text}
</Text>
<TouchableHighlight
style={styles.button}>
onPress = {this.lowercase}>
<Text style={styles.buttonText}>
Lowercase
</Text>
</TouchableHighlight>
</View> ); } 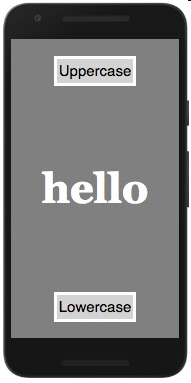
a. | export default class App extends Component { state =
{ text: 'hello',
}
uppercase = () => {
this.setState ({ text:
this.state.text.toUpperCase() })
}
lowercase = () => {
this.setState ({ text:
this.state.text.toLowerCase() })
} | c. | export default class App extends Component { state =
{ text: 'hello',
if
(text == uppercase) { this.setState
({ text:
this.state.text.toUpperCase() })
else { this.setState
({ text:
this.state.text.toLowerCase() })
} | b. | export default class App extends Component { state =
{ text: 'hello',
if
(text == uppercase) { this.setState
({ text:
this.state.text.toUpperCase() })
else if (text == lowercase) { this.setState
({ text:
this.state.text.toLowerCase() })
} | d. | export default class App
extends Component { state = {
text: 'hello', uppercase:
'HELLO', lowercase:
'hello' } |
|
|
15.
|
Assume the following state values have been set to create the output shown
below: export default class App extends Component { state =
{ miles:
0, season: 'Spring'
}  What code must be written inside
‘render()’ to create this layout where the values of ‘season’ and
‘miles’ can be updated?
a. | render() { return
( <View
style={styles.container}> <Text
style={styles.paragraph}>
Miles run in
</Text> <Text
style={styles.paragraph}>
{this.state.season}
</Text> <Text
style={styles.paragraph}>
{this.state.miles}
</Text> </View>
); } | c. | render() { return
( <View
style={styles.container}>
<Text style={styles.paragraph}>
Miles run in
</Text> <Text
style={styles.paragraph}>
miles
</Text> </View>
); } | b. | render() { return
( <View
style={styles.container}>
<Text style={styles.paragraph}>
Miles run in
{this.state.season}
</Text> <Text
style={styles.paragraph}>
{this.state.miles}
</Text> </View>
); } | d. | render()
{ return ( <View
style={styles.container}>
<Text style={styles.paragraph}>
Miles run in {this.state.season}
{this.state.miles}
</Text> </View>
); } |
|
|
16.
|
Answer the following question based on the phone screen shown below: 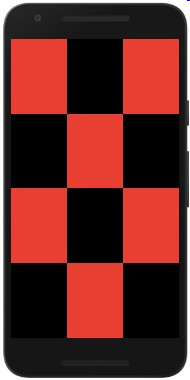 If the total height of the phone screen was 400 pixels, how tall is each red and
black box shown, assuming all boxes are the same height?
a. | 400 px | c. | 100 px | b. | 200 px | d. | 50 px |
|
|
17.
|
Answer the following question based on the phone screen shown below: 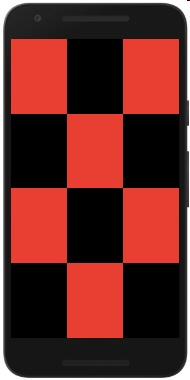 If screenWidth holds the value of the total width of the phone screen pictured,
what equation could be used to control the width of each box, if every box has an equal width?
a. | 3 | c. | screenWidth * 3 | b. | screenWidth/3 | d. | screenWidth/3 *
3 |
|
|
18.
|
Answer the following question based on the phone screen shown below: 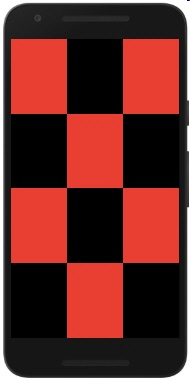 What aspect would need to be included somewhere in the style of this layout in
order to correctly display the boxes in the way depicted?
a. | alignItems: ‘center’ | c. | justifyContent:
‘center’ | b. | flexDirection:
‘column’ | d. | flexDirection: ‘row’ |
|
|
19.
|
Answer the following question based on the phone screen shown below: 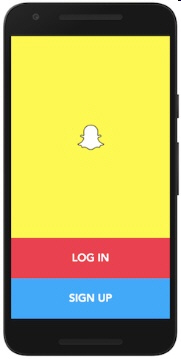 The height of the yellow section in the screen below is controlled by the equation
5*(screenHeight/7), where screenHeight is equal to the total height of the phone screen. If the blue
and red sections have equal heights, what equation would be used to control each of their
heights?
a. | 7*(screenHeight/5) | c. | screenHeight/7 | b. | screenHeight/2 | d. | 2*(screenHeight/7) |
|
|
20.
|
Answer the following question based on the phone screen shown below: 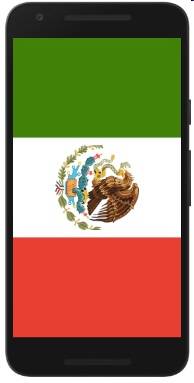 What information is necessary to include in the whiteStripe style in order to
correctly create the layout shown? 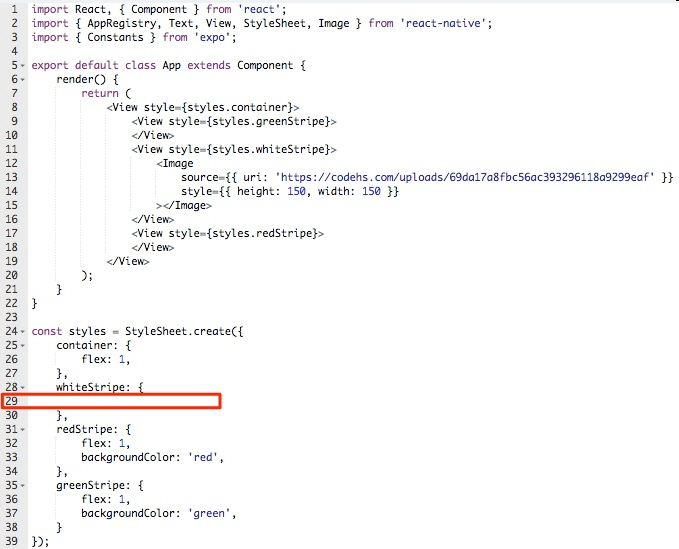
a. | backgroundColor: 'white', alignItems:
'center', justifyContent: 'center' | c. | alignItems:
'center', justifyContent: 'center' | b. | flex:
1, alignItems: 'center', justifyContent: 'center' | d. | flex: 1, backgroundColor:
'white', alignItems: 'center', justifyContent:
'center' |
|