Completion Complete each
statement.
|
|
1.
|
_______________________ are Unity’s default methods that run in a very
particular order over the life of a script.
|
|
2.
|
______________ is creating a reusable pool of objects that can be activated and
deactivated rather than instantiated and destroyed, which is much more efficient.
|
|
3.
|
What is the Build type for projects to be uploaded and embedded online?
|
|
4.
|
What is the operator used in C# to output the remainder or Modulus?
|
|
5.
|
________________ is an attribute that allows protected access to derived
classes.
|
Multiple Choice Identify the
choice that best completes the statement or answers the question.
|
|
6.
|
If you want to move the character up continuously as the player presses the up
arrow, what code would be best in the two blanks below: 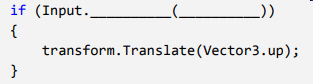
a. | GetKeyDown(UpArrow) | c. | GetKey(KeyCode.UpArrow) | b. | GetKeyHeld(Vector3.Up) | d. | GetKeyUp(KeyCode.Up) |
|
|
7.
|
Which of the following variable declarations observes Unity’s standard
naming conventions (especially as it relates to capitalization)? 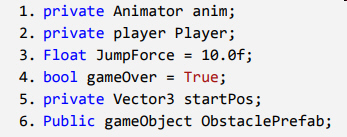
a. | 2 and 4 | c. | 3 and 6 | b. | 4 and 5 | d. | 1 and 5 |
|
|
8.
|
If you want to destroy an object when its health reaches 0, what code would be
best in the blank below? 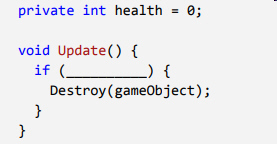
a. | health < 0 | c. | health < 1 | b. | health > 0 | d. | health.0 |
|
|
9.
|
Which comment would best describe the code below?
horizontalInput =
Input.GetAxis("Horizontal"); transform.Rotate(Vector3.up, horizontalInput);
a. | // Moves object up/down based on the the left/right arrow keys | c. | // Rotates around
the Z axis based on up/down arrow keys | b. | // Rotates around the Y axis based on
left/right arrow keys | d. | //
Rotates in an upward direction based on left/right arrow keys |
|
|
10.
|
What best describes the difference between the below images, where the car is in
the second image is further along the road? 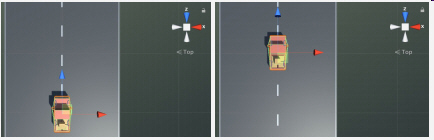
a. | The second car’s Y location value is higher than the first
car’s | c. | The second car’s Z location value is higher than the first
car’s | b. | The second car’s Transform value is higher than the first
car’s. | d. | The second
car’s X location value is higher than the first car’s |
|
|
11.
|
If you wanted a button to display the message, “Hello!” when a
button was clicked, what code would you use to fill in the blank? 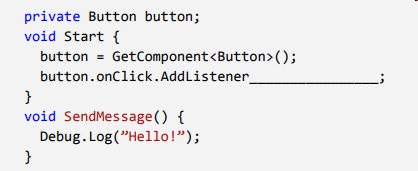
a. | (SendMessage); | c. | (SendMessage(string Hello)); | b. |
(SendMessage(Hello)); | d. | (SendMessage(“Hello”)); |
|
|
12.
|
The image below shows the preferences window that allows you to change which
script editing tool (or IDE) you want to use. Where would you click to choose an alternative code
editing tool? 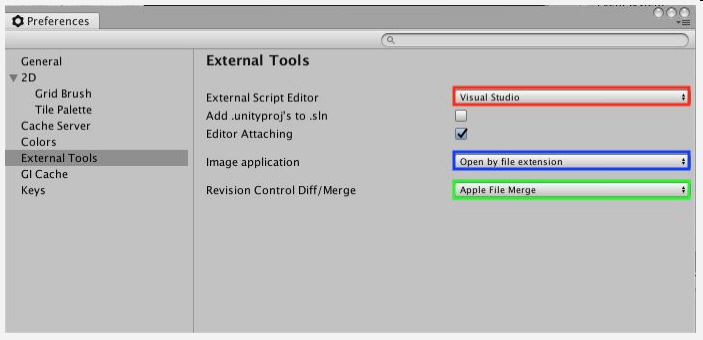
a. | The green box | c. | The red box | b. | The blue boc |
|
|
13.
|
The code below creates an error that says, “error CS1503: Argument 1:
cannot convert from 'UnityEngine.GameObject[]' to
'UnityEngine.Object'”. What could you do to remove the errors? 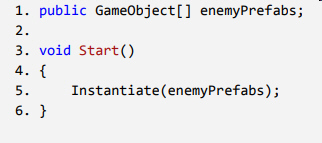
a. | Both On line 1, change “GameObject[]” to
“GameObject” and On line 5, change “enemyPrefabs” to
“enemyPrefabs[0]” together | e. | On line 1, change
“GameObject[]” to “GameObject” | b. | On line 3, change
“Start()” to Update()” | f. | Either On line 1, change
“GameObject[]” to “GameObject” or On line 5, change
“enemyPrefabs” to “enemyPrefabs[0]” | c. | Both On line 1,
change “enemyPrefabs” to “enemyPrefabs[0]” and On line 3, change
“Start()” to Update()” together | g. | On line 5, change “enemyPrefabs” to
“enemyPrefabs[0]” | d. | On line 1, change “enemyPrefabs” to
“enemyPrefabs[0]” |
|
|
14.
|
You’re trying to create some logic that will tell the user to speed up if
they’re going too slow or to slow down if they’re going too fast. How should you arrange
the lines of code below to accomplish that? 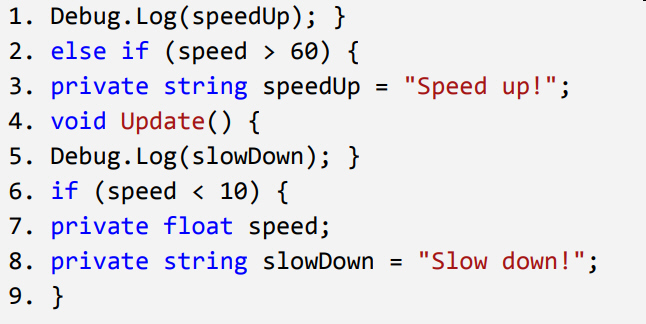
a. | 4, 6, 1, 2, 5, 9, 7, 8, 3 | c. | 7, 8, 3, 4, 6, 5, 2, 1,
9 | b. | 7, 8, 3, 4, 6, 1, 2, 5, 9 | d. | 6, 1, 2, 5, 7, 8, 3, 4, 9 |
|
|
15.
|
You’re trying to write some code that creates a random age between 1 and
100 and prints that age, but there is an error. What would fix the error? 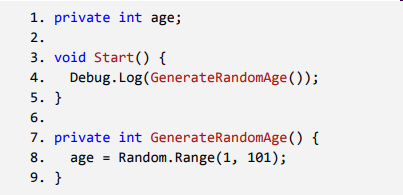
a. | Add the word “int” to line 8, so it says “int age =
…” | c. | Add a new line after line 8 that says “return age;” | b. | Change line 1 to
“private float age” | d. | On line 7, change the word “private” to
“void” |
|
|
16.
|
Which of the following follows Unity naming conventions (especially as they
relate to capitalization)? 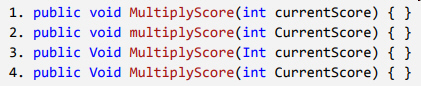
a. | Line 3 | c. | Line 2 | b. | Line 1 | d. | Line 4 |
|
|
17.
|
Which of the following statements about functions/methods are correct: 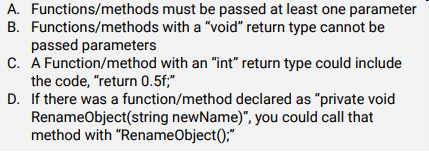
a. | B and C are correct | d. | Only B is correct | b. | None are correct | e. | A and B are correct | c. | Only D is
correct |
|
|
18.
|
Which of the following conditions properly tests that the game is NOT over and
the player IS on the ground 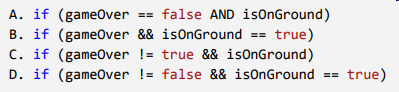
a. | Line B | c. | Line D | b. | Line C | d. | Line A |
|
|
19.
|
Given the animation controller / state machine below, which code will make the
character transition from the “Idle” state to the “Walk” state? 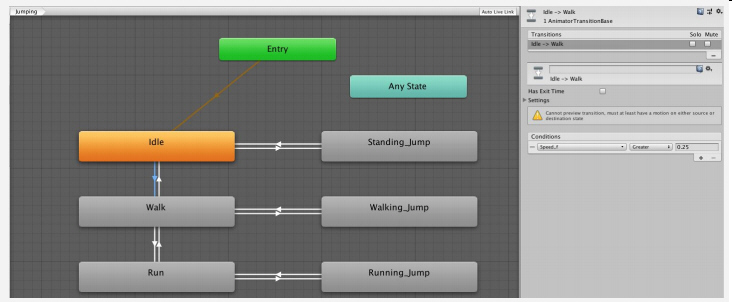
a. | setFloat(“Speed_f”, 0.3f); | c. | setInt(“Speed_f”,
1); | b. | setTrigger(“Speed_f”); | d. | setFloat(“Speed_f”,
0.1f); |
|
|
20.
|
You are trying to STOP spawning enemies when the player has died and have
created the two scripts below to do that. However, there is an error on the underlined code,
“isAlive” in the EnemySpawner script. What is causing that error? 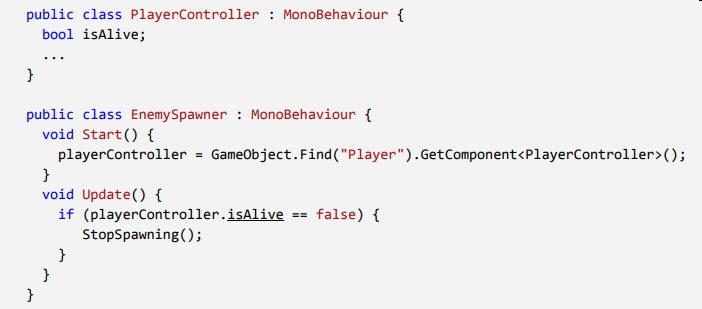
a. | The “p” should be capitalized in
“playerController.isAlive” | c. | The if-statement cannot be in the Update method | b. | “isAlive” must start with a capital “I”
(“IsAlive”) | d. | The
“bool” in the PlayerController class needs a “public” access
modifier |
|
|
21.
|
You are trying to assign the powerup variable in the inspector, but it is not
showing up in the Player Controller component. What is the problem? 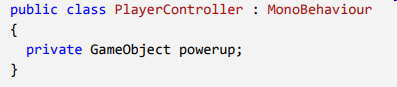
a. | The PlayerController class should be private instead of public | c. | The powerup
variable should be public instead of private | b. | You cannot declare a powerup variable in the
Player Controller Script | d. | You cannot assign GameObject type variables in the
inspector |
|
|
22.
|
If you have made changes to a prefab in the scene and you want to apply those
changes to all prefabs, what should you click? 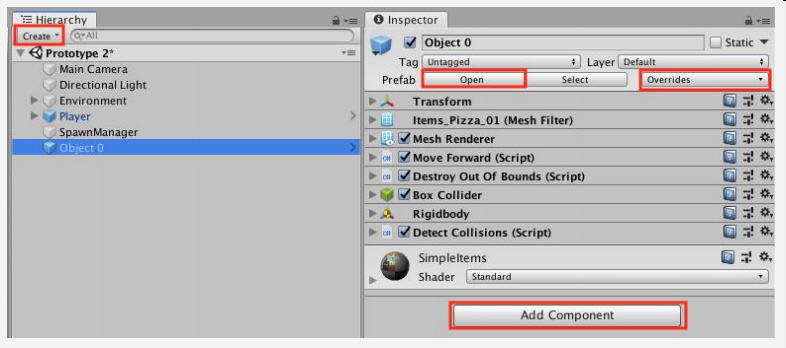
a. | The “Open” button at the top of the Inspector | c. | The
“Create” drop-down at the top of the Hierarchy | b. | The
“Overrides” drop-down at the top of the Inspector | d. | The “Add Component” button at the
bottom of the Inspector |
|
|
23.
|
Which of the following do you think makes the most sense for a simple movement
state machine? 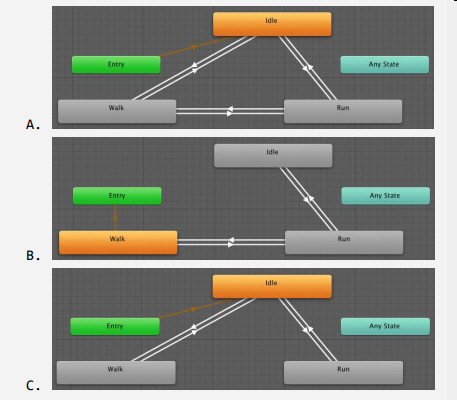
a. | Image B | c. | Image C | b. | Image A |
|
|
24.
|
Which comment best describes the following code? 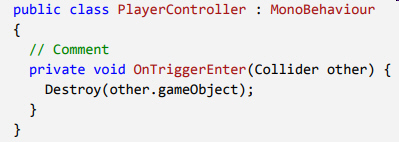
a. | // If enemy collides with another object, destroy the object | c. | // If player
collides with another object, destroy player | b. | // If player collides with a trigger, destroy
trigger | d. | // If player
collides with another object, destroy the object |
|
|
25.
|
You are trying to create a new method that takes a number and multiplies it by
two. Which method would do that? 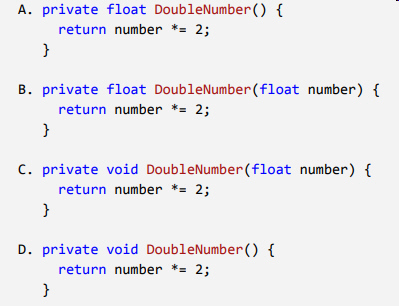
a. | Method D | c. | Method B | b. | Method C | d. | Method A |
|
|
26.
|
Read the Unity documentation below about the OnMouseDrag event and the code
beneath it. What will the value of the “counter” variable be if the user clicked and held
down the mouse over an object with a collider for 10 seconds? 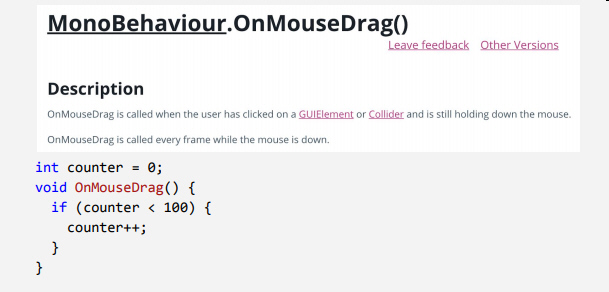
a. | 1 | d. | 100 | b. | A value over 100 | e. | 0 | c. | 99 |
|
|
27.
|
You are trying to randomly spawn objects from an array. However, when your game
is running, you see in the console that there was an “error at
Assets/Scripts/SpawnManager.cs:5. IndexOutOfRangeException: Index was outside the bounds of the
array.” Which line of code should you edit in order to resolve this problem and still retain
the random object functionality? 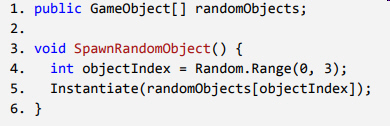
a. | Line 5 | c. | Line 3 | b. | Line 1 | d. | Line 4 |
|
|
28.
|
The code below produces the error, “error CS0029: Cannot implicitly
convert type 'UnityEngine.GameObject' to 'UnityEngine.Rigidbody'”. What
could be done to fix this issue? 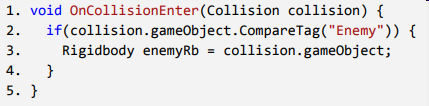
a. | . On line 3, add “.GetComponent<Rigidbody>()” before the
semicolon | c. | On line 3, delete “.gameObject” | b. | On line 1, change
“collision” to “Rigidbody” | d. | On line 2, change “gameObject” to
“Rigidbody” |
|
|
29.
|
Which of the following follows Unity’s naming conventions (especially as
it relates to capitalization)? 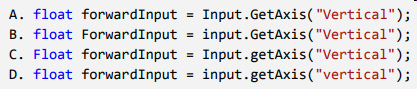
a. | Line C | c. | Line A | b. | Line D | d. | Line B |
|
|
30.
|
You run your game and get the following error message in the console,
“NullReferenceException: Object reference not set to an instance of an object”. Given the
image and code below, what would resolve the problem? 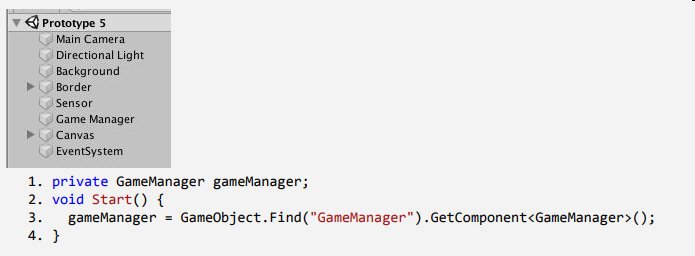
a. | On Line 1, rename “GameManager” as “Game
Manager” | c. | In the hierarchy, rename “Game Manager” as
“GameManager” | b. | On Line 3, remove the GetComponent
code | d. | In the hierarchy,
rename “Game Manager” to “gameManager” |
|
|
31.
|
By default, what will be the first state used by this Animation
Controller? 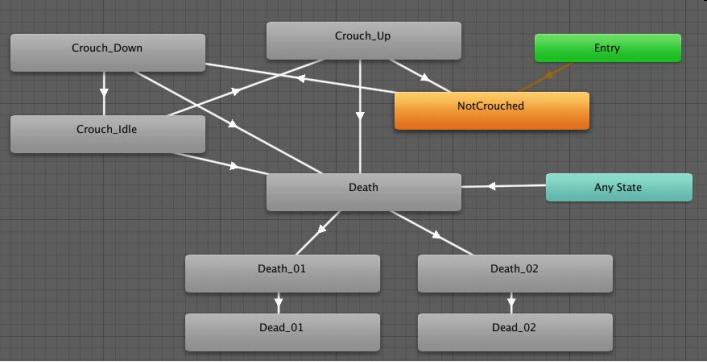
a. | “Death” | c. | “NotCrouched” | b. | “Any
State” | d. | “Crouch_Up” |
|
|
32.
|
Which of these is the correct way to get a reference to an AudioSource component
on a GameObject? 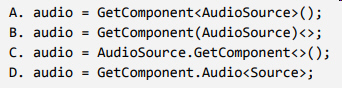
a. | Line D | c. | Line C | b. | Line A | d. | Line B |
|
|
33.
|
Read the documentation from the Unity Scripting API and the code below. Which of
the following are possible values for the randomFloat and randomInt variables? 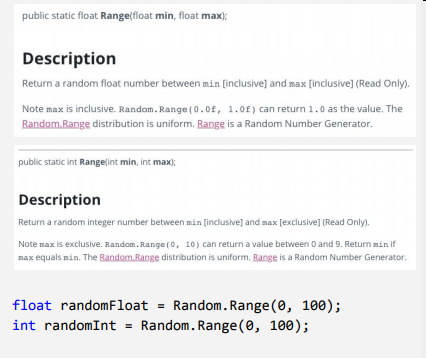
a. | randomFloat = 0.0f; randomInt = 50.5; | c. | randomFloat = 50.5f; randomInt =
100; | b. | randomFloat = 100.0f; randomInt = 100; | d. | randomFloat = 100.0f; randomInt =
0; |
|
|
34.
|
Which of the following is the correct way to declare a new List of game objects
named “enemies”? 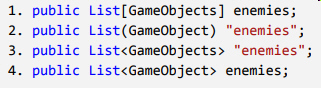
a. | Line 2 | c. | Line 4 | b. | Line 3 | d. | Line 1 |
|
|
35.
|
Look at the documentation and code below. Which of the following lines would NOT
produce an error? 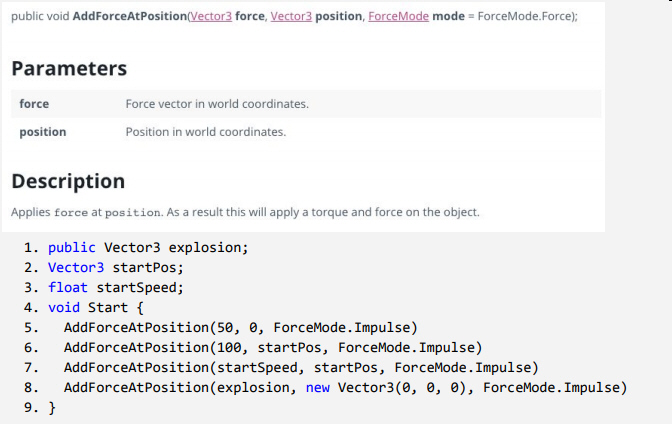
a. | Line 7 | c. | Line 5 | b. | Line 6 | d. | Line 8 |
|
|
36.
|
Based on the code below, what will be displayed in the console when the button
is clicked? 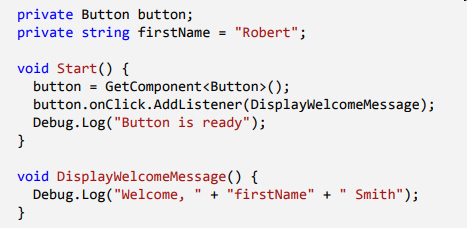
a. | “Welcome + Robert + Smith” | c. | “Welcome, firstName
Smith” | b. | “Button is ready” | d. | “Welcome, Robert
Smith” |
|
|
37.
|
Which of the following lines of code is using standard Unity naming
conventions? /* a */ Public Float Speed = 40.0f; /* b */ public float Speed = 40.0f; /* c */
Public float speed = 40.0f; /* d */ public float speed = 40.0f;
a. | Line B | c. | Line C | b. | Line A | d. | Line D |
|
|
38.
|
Which of the following is most likely the condition for the transition between
“Run” and “Walk” shown below? 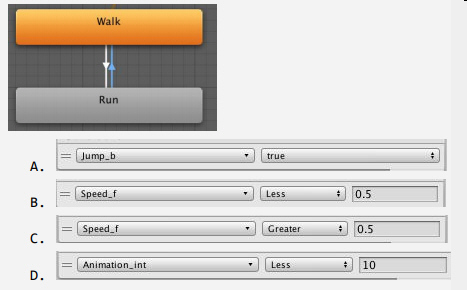
a. | Animation_int is Less than 10 | c. | Jump_b is true | b. | Speed_f is Greater
than 0.5 | d. | Speed_f is Less
than 0.5 |
|
|
39.
|
When you run a project with the code below, you get the following error:
“NullReferenceException: Object reference not set to an instance of an object.” What
is most likely the problem? 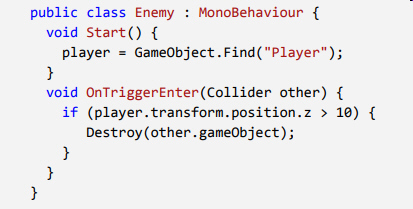
a. | There is no object named “Player” in the scene | c. | . The Enemy object
does not have a Rigidbody component | b. | The “Start” method should actually
be “Update” | d. | The
Player object does not have a collider |
|
|
40.
|
Read the documentation from the Unity Scripting API below. Which of the
following is a correct use of the InvokeRepeating method? 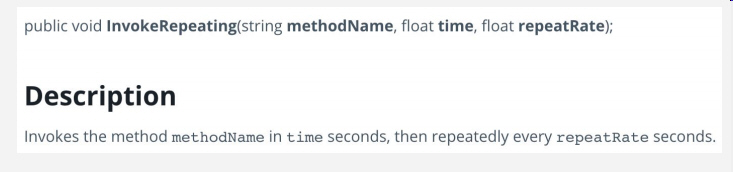
a. | InvokeRepeating(“Spawn, 0.5f, 1.0f”); | c. | InvokeRepeating(“Spawn”, 0.5f, 1.0f); | b. | InvokeRepeating(0.5f, 1.0f, “Spawn”);
| d. | InvokeRepeating(“Spawn", gameObject,
1.0f); |
|
|
41.
|
In what order do you put the words when you are declaring a new
variable? public float speed = 20.0f;
a. | variable name] [data type][variable name] [data
type] | c. | [data type] [access modifier][variable name]
[variable value | b. | [access modifier] [data
type][variable name] [variable value] | d. | [data type] [access
modifier][variable value] [variable name] |
|
|
42.
|
If it says, “Hello there!” in the console, what was the code used to
create that message?
a. | Debug.Log(Hello there!); | c. | Debug.Log("Hello
there!"); | b. | Debug.Console(“Hello there!”); | d. | Debug(“Hello
there!”); |
|
|
43.
|
What is true about the following two lines of code? 
a. | They will both move an object in the same direction | c. | They will both move an object along
the same axis | b. | They will both move an object at the same speed | d. | They will both rotate an object, but along
different axes |
|
|
44.
|
The following message was displayed in the console: “Monica has 20
dollars”. Which of the line options in the PrintNames function produced it? 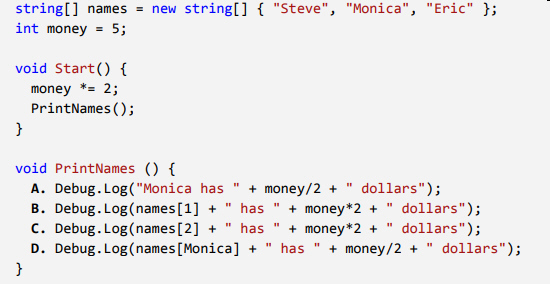
a. | Option B | c. | Option A | b. | Option D | d. | Option C |
|
|
45.
|
Which comment best describes the code below? 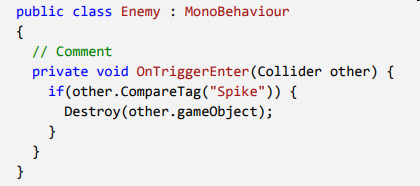
a. | // If the enemy collides with a spike, destroy the enemy | c. | // If the player
collides with an enemy, destroy the enemy | b. | // If the enemy collides with a spike, destroy
the spike | d. | // If the player
collides with a spike, destroy the spike |
|
|
46.
|
The code below produces “error CS0029: Cannot implicitly convert type
'float' to 'UnityEngine.Vector3'”. Which of the following would remove the
error? 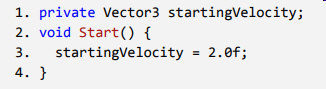
a. | None of the options | c. | On line 1, change “Vector3” to
“float” | b. | Either On line 1, change “Vector3”
to “float” OR On line 3, change “=” to “+” | d. | On line 3, change “=” to
“+” |
|
|
47.
|
Which of the following variables would be visible in the Inspector? 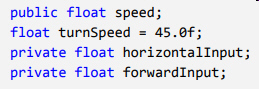
a. | turnSpeed | c. | speed | b. | horizontalInput &
forwardInput | d. | speed &
turnSpeed |
|
|
48.
|
Which Unity window contains a list of all the game objects currently in your
scene?
a. | Project window | c. | Hierarchy | b. | Inspector | d. | Scene view |
|
|
49.
|
If there is a boolean in script A that you want to access in script B, which of
the following are true: 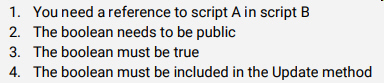
a. | 1, 2, and 3 only | d. | 3 and 4 only | b. | 1 and 2 only | e. | 1 only | c. | 2 and 3
only | f. | All are
true |
|
|
50.
|
What is a possible value for the horizontalInput variable? 
a. | “Right” | c. | -10 | b. | Vector3.Up | d. | 0.52 |
|